The effect i show up till now are all discontinuous effect that can be easily create using CSS and jQuery. Some people may wonder how continuous sliding effect is being achieved. In this tutorial, i will demonstrate the concept and a plugin which i build immediately during this tutorial on how to simultaneously pull out the containers in the easily way. The demo can be view at the bottom of this post.
Concept
The concept for continuously sliding effect is really not that difficult. Imagining you have a photo frame and a long list of photo arranged in a straight row. The list is placed behind the photo frame and you started to pull it accordingly to display a new photo within the frame. Anything outside the frame is being blocked which restrict the display only within the frame itself. This way you will see a continuously slide effect being displayed.
CSS
From the description above, it is clear that css is required to assist on building the effect (every type of effect cannot escape the demon hand of CSS! RAWR!). And below listed the required CSS rule from the description written above.
- float: left - to flow all containers in a straight row
- position: relative - so that the container can be hidden in IE and FF
- overflow: hidden - we want to hide all other element outside the frame
- margin-left or left: - this is where we adjust the element so that it get pull to the left or right within the frame
HTML
On the description above, we will have a rough idea how many containers are required to built the effect and plugin. The following list down the approximate container required.
- Main Frame
- container to contain x element
- the x number of elements in the frame
- next button
- right button
Everything is put it simple so that we have a clear idea how this is being done. The reason why we need the extra container to contain the x element is because the element WILL NOT float side by side if you do not define a length that is big enough for all elements to go side by side.
Coding
This part always get a bit confusing when there are piece and pieces of code flying around the place. But i will try my best to explain what is happening in here.
On the HTML page, i have allocated the following containers as describe above.
<div id='frame'> <div id='container'> <div class='box' id='box1'></div> <div class='box' id='box2'></div> <div class='box' id='box3'></div> <div class='box' id='box4'></div> </div> <div id='prev'></div> <div id='next'></div> </div>
It should be clear that there is 3 main containers which are the frame,next and right button. And within the frame there is another container which contain the 4 element that we will be interested to slide them around. The reason why both navigation button are within the frame so that the button will always be within the container.
On the CSS external script, the below listed out all the necessary elements that we mention on the CSS section.
body{ margin: 0 auto; /*align the display at the center*/ text-align: center; /*align the display at the center*/ } div#frame{ margin: 0 auto; /*align the frame at the center*/ width: 50em; /*frame width*/ height: 25em; /*frame height*/ border: #000 solid 10px; /*frame border*/ position: relative; /*position relative so that it gets hide in IE*/ overflow: hidden; /*hide all overflow element out of the frame*/ } div#container { position: relative; /*position relative so that it gets hide in IE*/ width: 200em; /*the width must be big enough so that all elements can align side by side*/ height: 25em; /*container height*/ } div.box { float: left; /*float each element side by side*/ width: 50em; height: 25em; position:: relative; } div#box1 { background-color: #66A666; } div#box2 { background-color: #E37373; } div#box3 { background-color: #B2B2B2; } div#box4 { background-color: #A51515; } div#next { width: 3em; height: 5em; top: 50%; /*align it vertically center*/ margin-top: -2.5em; /*align it vertically center*/ right: 0em; position: absolute; background: transparent url(../images/next.png); } div#prev { width: 3em; height: 5em; left: 0em; top: 50%; /*align it vertically center*/ margin-top: -2.5em; /*align it vertically center*/ position: absolute; background: transparent url(../images/prev.png); }
If you wonder how the left and right button is aligned on center, you can see the explanation at How to align center on the screen when using position absolute with CSS
i have indicated the comment on the code itself so that it can be self explain instead. After all of these settings, you should be able to get something like this.
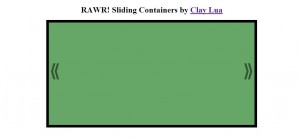
Look pretty decent right? Now come the real code in jQuery. So we want to pull to the next element to the right to simulate continuously sliding, we will attach an event handler to both next and previous button. Using the animate() function in jQuery, this will be a piece of cake.
$(function(){ var i = 0; $(this).click(function() { if(this.id==op.prevImageID.replace("#","")) { i++; if(i > 0) i=(0-(op.noOfContainer-1)) } else if(this.id==op.nextImageID.replace("#","")) { i--; if(i<(0-(op.noOfContainer-1))) i=0; } $("div#container").animate({marginLeft: i*op.containerW+"em"},op.duration); });
The first sentence in the code indicate that if previous button is pressed, we add the counter and check whether it is more than the available container. If the counter has go pass the available containers, we will push it to the last container available. We did the same for the next button too! but in descending order. Finally, we animate the scrolling according to the the given counter with the size of each element.
Plugin
I really do not know whether there is a need for such plugin since its really quite simple. But i will still provide it like i said previously. So with the explanation above, i will refractor the code a bit so that it is flexible enough to become a plugin.
Parameter
- prevImageID: previous button image id
- nextImageID: next button image id
- noOfContainer: number of container
- containerW: width of container
- duration: duration of animation
in order to change the display, just change the css code in ini.css. No hardcoded code in the javascript itself. There can be more flexibility in the future of this plugin which i will place in more feature if i get the time. If you would like to support me, you can always throw in some bucks for the maintance of this plugin.