This is another method for jQuery zoom effect that you can apply. Unlike the previous tutorial on zoom effect which is pretty simple and straight forward, this approach tend to be more complex. The previous method mention has
jQuery
Tutorial: jQuery Fold Effect Concept And Explanation
In this tutorial, i will explain the concept and ways of making a fold effect in jQuery. Such effect can be apply to different context such as image gallery or even just add on effect for your design work.
Concept
Depending on whether you wish to fold away your content or you wish to fold out your content. Folding in your content will be to pull up the content and slide it outward while folding out your content will be to push your content out and slide it downwards. There are two way of achieving this effect. One is to use the viewport technique which will lengthy your code and complex your stuff but generally give you a better visualize effect than the next effect. The next effect that can achieve this with only few lines of code is typically using CSS and animate function in jQuery.
Coding
On the HTML part, you will most likely will only required the following set of div block
<div class='image' id='box1'></div> <div class='image' id='box2'></div> <div class='image' id='box3'></div> <div class='image' id='box4'></div>
On the CSS coding, you will most likely need the following
body{ margin: 0 auto; /*align the header at the center*/ text-align: center; /*align the header at the center*/ } div.image { width: 600px; /* width of each container*/ height: 350px; /* height of each container*/ position: absolute; /* instructure each container to obey the position absolutely*/ float: left; /* float all the container so that they overlapped each other*/ left: 27%; /* align them to the center of the screen */ z-index: -1; } div#box1 { background: #66A666 url('../images/blue.png'); } div#box2 { background: #E37373 url('../images/green.png'); } div#box3 { background: #B2B2B2 url('../images/orange.png'); } div#box4 { background: #A51515 url('../images/red.png'); }
The above codes are similar to the opening door effect tutorial i did previously.
On the jQuery coding,
$(function(){ var i = -1; $('div.image').click(function(){ $(this) .animate({height: 50+'px'}, 500) .animate({width: 0+'px'}, 500, function(){$(this).css('z-index', i); $(this).css({height: 350+'px', width: 600+'px'});}); i--; }); })
this is all you required. What i did is basically add an event handler to each div block and perform an animate function to adjust the height of the effect to a certain visible amount and slide the height to 0 once the height animate has completed which will gives us a nice fold effect. Once the fold effect has been completed, i push the image back to the queue by setting its z-index and return its original size. This way is much better than using a view port which will block the next image and create a not very impressive fold effect.
You can visit the demo site for this fold effect tutorial. The tutorial files can be retrieve from jquery-fold-effect-tutorial
Tutorial: How to make your own opening and closing door effect with jQuery
The kind words from some of you guys really makes me wanted to write more advance tutorial but i really have limited time. Nonetheless, i will try my best to write at least 1 tutorial in the most easiest and detail form as much as i can. In this tutorial, i will show you a more advance trick to perform a opening/closing door effect. This tutorial will deal with viewport to perform such effect and may get a little confusing. The demo is as usual at the bottom of the tutorial. If you would like to view the demo first before reading, please proceed to the bottom of this tutorial instead.
Concept
Imagine you have a sliding door, one left and one right. In order to open/close this particular door, we have to pull or push both door so that it will open or close completely. This is the usual case for a door to open and close which required two image of door left and right. How can this be done? The concept behind this technique is not similar to the zoom tutorial i did previously. But let's just concentrate on what is a viewport. A viewport is just a viewable area on the screen to the user, anything outside the viewport is considered unseen by the user. Using viewport, we will try to hide the two sliding door left and right outside the viewport and when the user clicks on the image, the door will automatically be called in and closed the door to create a nicely done closed door effect. The opening door effect will be exactly the same with the additional step to open the door after the door is closed.
CSS and HTML
I believe the CSS and HTML structure should be the same as the shuffle effect tutorial i wrote previously.
Coding
The coding part may get a bit confusing for the viewport technique. But i will try my best to cover it with simplicity as much as i can. If there is any doubt you may have you can really comment below and i will try to reply you within the day if possible. On the CSS Coding,
body{ margin: 0 auto; /*align the header at the center*/ text-align: center; /*align the header at the center*/ } div.image { width: 600px; /* width of each container*/ height: 350px; /* height of each container*/ position: absolute; /* instructure each container to obey the position absolutely*/ float: left; /* float all the container so that they overlapped each other*/ left: 27%; /* align them to the center of the screen */ z-index: -1; } div#box1 { background: #66A666 url('../images/blue.png'); } div#box2 { background: #E37373 url('../images/green.png'); } div#box3 { background: #B2B2B2 url('../images/orange.png'); } div#box4 { background: #A51515 url('../images/red.png'); }
Notice that everything above are images and if images are not displayed yet, a color will be shown instead. Other important stuff are self explained on the comment i wrote in the code. For the HTML structure,
<div class='image' id='box1'></div> <div class='image' id='box2'></div> <div class='image' id='box3'></div> <div class='image' id='box4'></div>
There will only be the container in the HTML structure similar to the shuffle effect tutorial. Supposely, it should have more than just 4 block of div as we need a viewport for each container. But for simplicity, i will just write 4 block here and leave all the complex stuff with jQuery. On the jQuery side,
//below here preload the image in the simplest form $(function(){ for(i = 1; i < 5;i++) { var img = new Image(); img.src = 'images/images/d_left_'+i+'.png'; $(img).load(); } var i = -1; //deep of the image var no = 0; //image number //attach event handler on each container/image $('div.image').click(function(){ var Obj = $(this); no++; if(no > 4) no = 1; // viewport structure Obj.wrap(' <div id='viewport'></div> '); Obj.css({left: 0+'px'}); $('#viewport').css('overflow','hidden'); $('#viewport').width(Obj.width()); $('#viewport').height(Obj.height()); $('#viewport').css('left','27%'); $('#viewport').css('position','absolute'); // left door structure $('#viewport').append(' <div class='GrpEffectDiv' id='doorLeft'/>'); $('#doorLeft').css('position', 'absolute'); $('#doorLeft').css('background', '#000 url('images/images/d_left_'+no+'.png')'); $('#doorLeft').width(Obj.width()/2); $('#doorLeft').height(Obj.height()); $('#doorLeft').css('left', '-'+300+'px'); //right door structure $('#viewport').append(' <div class='GrpEffectDiv' id='doorRight'/>'); $('#doorRight').css('position', 'absolute'); $('#doorRight').css('background', '#000 url('images/images/d_right_'+no+'.png')'); $('#doorRight').width(Obj.width()/2); $('#doorRight').height(Obj.height()); $('#doorRight').css('left', 600+'px'); // left door animation $('#doorLeft') .animate({left: 0+'px'},1000, function(){ Obj.css('z-index', i); $(this).remove(); }); //right door animation $('#doorRight') .animate({left: 300+'px'},1000, function(){ $(this).remove(); Obj.css({left: '27%'}); $('#viewport').replaceWith(Obj); }); i--; }); })
Seriously, looking at the code i am starting to wonder whether i will be able to explain it in a simple term. Anyway, what the above code done is as follow,
- create a viewport around the container and became the parent of the container
- create the left door and append into the viewport ( doors became neightbour of the container)
- create the right door and append into the viewport
- animate both left and right door to close the door
- remove both door and subsituate the viewport back to the container with the original settings
I have also commented the code above so that you are clear what i am doing on the code itself. So how does the viewport be created by the code above? If you have read my sliding tutorial previously, it is similar to the frame concept, where there is a frame (viewport) to cover the outside of the viewport. If i remove the most important thing for the viewport to work which is
$('#viewport').css('overflow','hidden');
You will see that the left and right box is standing by on the right and left side ready to charge at the container to close the door.
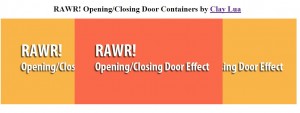
Notice the right and left image which i made? These are the door of the next image, this way we can create a very nice effect as shown in the demo
.
Improvement
There maybe people who will disagree with this tutorial that all the styling is placed on the jQuery script itself when we can actually just add a class on an external script and give the class to the div block object itself instead. You can definitely do that to simplify the efficiency and flexibility of the code. The purpose why it is done this way is to avoid any complexity on the CSS section. Personally, it will just confuse me going to look up and down on the post to feature out what i am doing. So i place it in a chunk of code indent it nicely for me and everyone to understand.=) The other thing you can do to improve the above code is to apply chaining which i always did in a whole straight line. Imagine if i do it in my tutorial? won't be that nice to read isn't it? Another thing is the preloader in this tutorial, it is just pure load image and may not do as well as other great pre loader but it basically how preloader work in jQuery. Thus, you may see black door on the first time during your loading instead of the image of the door until it cache the image to your browser that it! =X You can do this for gate closing and opening effect as well, we just have to do it on the top and bottom instead of left and right. Anyway, hope you like this tutorial as well =)
File
You can find the tutorial files by saving the page on the demo site. But if you feel lazy, you can download from jquery-closing-opening-door-effect
Tutorial: How to create your own inner fade effect with jQuery
Just when i though i have completely writing all the effect tutorial available, i neglected inner fade effect that has been around for quite some time. Maybe it look exactly like fade effect in jQuery and didn't really notice the differences. Just to explain what does inner fade effect differ from normal fade effect in jQuery, inner fade effect will display the combination of both images when the first image gets fade in. This produces an asynchronous effect of image being displayed together. On the other hand, normal fade effect produces fade in an synchronous way when one fade ended the other fade occurs. The demo can be view at the bottom of this tutorial.
Concept
The concept for an inner fade is much troublesome than a normal fade effect in jQuery. A normal fade effect in jQuery will only required the fadeIn,fadeOut or fadeTo method to be called by the chaining process. Inner fade effect will require an additional step which is to arrange the images into a stack. Similar to the shuffle concept but we will just have to use the built-in feature of jQuery, animate to complete this tutorial. Imagine you have a deck of cards, each touch will make the card slowly fade till disappear and display the next card. So, what happen to the disappeared card? Similarly, we set the displayed index to a lower value and hide it behind of the deck of cards. So when the deck of cards completely disappeared, it will fade to the start card again!
CSS and HTML
Similarly, it will be exactly the same as the shuffling tutorial.
Code
The CSS and HTML code will be pretty much the same as the shuffle effect tutorial. The only differences we will see in this tutorial compare to the shuffle effect tutorial is the jQuery code used to perform the task.
$(function(){ var i = 0; $('div.card').click(function(){ $(this) .animate({opacity: 0}, 1000, function(){$(this).css("z-index", i)}) .animate({opacity: 1}, 1000); i--; }); });
Notice that i did not use fadeTo method because the z-index command takes longer than the next animation to be display and cause the whole inner fade to fail. Thus, i use animate function instead and instruct jQuery to immediately set the z-index to i depth where i is the variable to keep track of the deepness of the container. This way i won't have to worry about the next animation coming forward quicker than the z-index rule. The other reason is that it seems buggy to use fadeTo function during this tutorial. Since, animate function work perfectly i will just stick to that then. From the demo page, you can notice that sometimes the colors are being combined and turn to some other color although there are only 4 colors available! This is the differences between inner fade and the normal fade. You can download the tutorial file at jquery-inner-fade-effect
Tutorial: How to make your own shuffle effect with jQuery
i think after you have read most of my effect tutorial on jQuery, you will notice that effect is just playing around with CSS. Nonetheless, without jQuery help using pure JavaScript to achieve the same result will really take a lot of time than the usually time we spend on jQuery. Anyway, back to the topic that i am going to write. In this tutorial, i will demonstrate another very simple concept to shuffle your containers or images. I believe this should be the last effect available in the market currently. Most of the effect i should have covered them up.
Tutorial
i find this way of writing tutorial is easy for me to understand and its much more neat and clearer compare to my other tutorial which is quite a mess. So i will continue using such structure to write my tutorial as much as possible. If you have any feedback or improvement that i can make, please send me an email or just leave a comment. =)
Concept
Let's imagine you have a set of poker card. The poker cards should be shown on the screen in a z-axis way. When we shuffle, we take the highest card, pull it out and placed at the back (Sound like a first in last out concept to me). Let's assume each container is a card. We will have to stack the card together in a z-axis row in order to simulate the pattern of a poker card deck. Well, since its programming we can really just have 2 containers and placed the image on the second level so that when the first image is being shuffled down, the second level image is being displayed during the shuffling of the first card. But let's not do such complex stuff, we are doing a simple tutorial right? Opps.
CSS
from the description in the concept section, the necessary and required CSS rule to apply on the containers are most probably something that can help us stack n containers together. Below listed the approximate rule required for shuffle effect to work.
- position: absolute - so that every container in the deck has a MUST obey position
- z-index: x - so that every element is being position in an z-axis level
- top: x em - the vertical position of the container
- left: x em - the horizontal position of the container
- float: left - we float all element so that they are no longer on the ground
where x is an integer value. Please take note that if you do not provide a type for your top and left, it may post a problem later when you try to shuffle your containers. Another thing to take note, z-index don't take in types like em or px, it is a pure number without type.
HTML
with the CSS rule declared, its time to setup our structure for each container. The structure of the container is fairly easy to setup. We just need a set of n containers in order to display different container during shuffling.
Coding
This part always get a bit confusing when there are piece and pieces of code flying around the place. But i will try my best to explain what is happening in here.
On the HTML page, i have allocated the following containers as describe above.
<div class='card' id='box1'></div> <div class='card' id='box2'></div> <div class='card' id='box3'></div> <div class='card' id='box4'></div>
On the CSS external script, the amount of code required is also smaller compare to other tutorial since there is only 1 type of container we are dealing in this tutorial. The stylesheet is as follow,
div.card { width: 25em; /* width of each container*/ height: 20em; /* height of each container*/ position: absolute; /* instructure each container to obey the position absolutely*/ float: left; /* float all the container so that they overlapped each other*/ left: 34%; /* align them to the center of the screen */ border: #000 solid 10px; /* give it a border to make it look nice*/ } div#box1 { background-color: #66A666;/*green*/ } div#box2 { background-color: #E37373;/*pink*/ } div#box3 { background-color: #B2B2B2;/*grey*/ } div#box4 { background-color: #A51515;/*red*/ }
There is something interesting in here that you might want to know, when we set position of a div tag, the z-index property is being set to auto in default. Thus, the last container in our example(box4) will be the one display on the top of the stack. Unless we overwrite the z-index css properly. This is what we will see after we have our structure and CSS done.
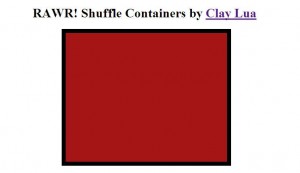
IT IS JUST A BOX! Definitely, this is a box alright. Other containers are behind the container shown on the image. Notice that the last container on the HTML structure is being displayed instead of the first container? This is the reason why z-index is important later when shuffling the containers.
Lastly, we will be on our way to tackle jQuery coding after we have complete the structure required for our shuffle effect. So what i will be going to do is to attach an event handler on each container. Once the event is being triggered, we will animate the top object to the right and pull it back down the queue by setting it z-index to a lower value. The code is presented below,
$(function(){ var i = 0; $('div.card').click(function(){ $(this) .animate({left: 15+'%', marginTop: 2+'em'},500, 'easeOutBack',function(){i--;$(this).css('z-index', i)}) .animate({left: 38+'%', marginTop: 0+'em'},500, 'easeOutBack'); }); });
The code done as what i instructed. Igive a variable i to hold the current level of z-index. adding event handler to all of my elements, once an event occurs, i will move the call object to the left and bottom 2em with the easing ability of easeOutBack from the jQuery plugin made available by George. After the animation has completed, i set the z-index of the container to a lower level and pull it back in with another animation. And this will give you a nice shuffle effect as shown in the demo. You can download the tutorial file at jquery-shuffle-effect
That is all i have for you in this tutorial hope you enjoy this! I sure did! Cheers~